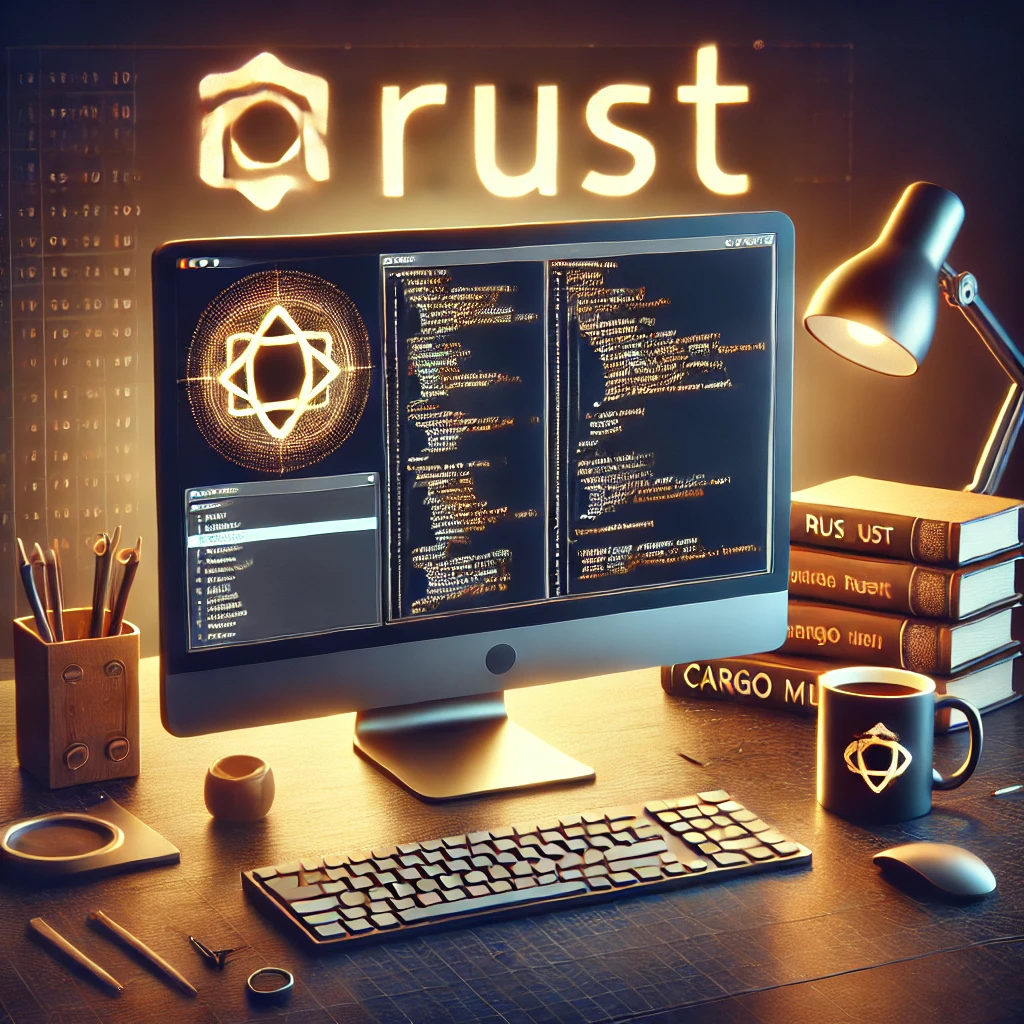
If this is the first time you are using Rust you will have to install Rust on your Windows machine.
To install see this link – https://www.rust-lang.org/tools/install
To install Rust on Windows subsystem for Linux, use this shell command-
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Check the version of Rust-
rustup --version
Check the version of Rust compiler
rustc --version

To update the Rust, use following command-
rustup update
Create a main.rs file and add code to print a string
fun main(){
println!("Hello World!")
}
Compile using rust compiler
rustc main.rs
This should create a pdb and exe file
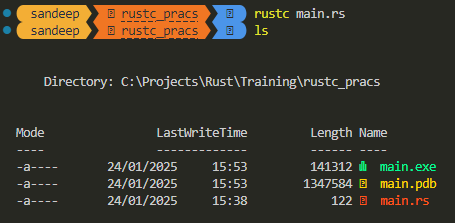
Execute the main.exe file and this should print the string

Create a Rust project using Cargo
Cargo is a build and package manager.
- Helps to manage dependecies for repetable builds
- Downloads and builds external libraries
- calls rustc with correct parameters
- Included with standard rustup installation
For creating a new Rust project using Cargo package manager-
cargo new first_rust_prj
Tip – The name of the project should be snake case. Example this will give a warning. Atlhough this will create a project.
cargo new FirstRustPrj //Warning
Creating binary (application) `FirstRustPrj` package
warning: the name `FirstRustPrj` is not snake_case or kebab-case which is recommended for package names, consider `firstrustprj`
This should create following folder structure with main.rs and Cargo.toml (configuration) file.
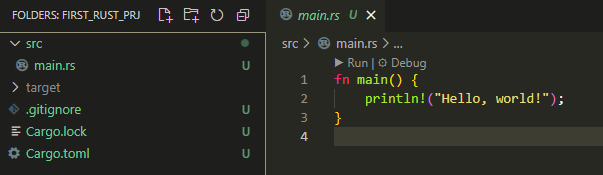
TOML – Tom’s Obvoius Minimal Language.
Alternatively, you can create a project without using Cargo. Create a src directory and create appropriate Cargo.toml namually. To create toml file use init command
cargo init
Building and Running Cargo project
Build the Cargo project using following command-
cargo build
To compile and run directly from the project folder, use following command-
cargo run

Error compiling the project-
error: linker link.exe
not found
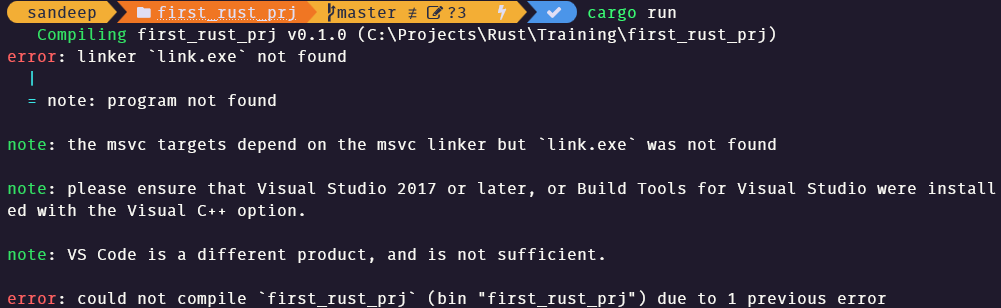
So the pre-requisite for windows machine is to have MS C++ build tools. Install the smae from here. It should be around 5 GB.
https://visualstudio.microsoft.com/visual-cpp-build-tools
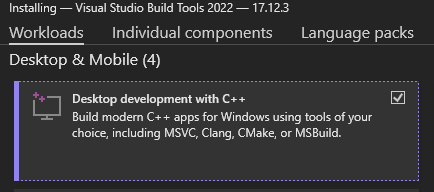
Now this should run the project –

To check the code without producing an executable use follwoing command-
cargo check
Building for Release
Use following command when project is ready for release-
cargo build --release
IDE to develop rust-
Visual Studio Code
Install Visual Studio Code. Then install rust-analyzer for code auto completion, hints and code actions – Link
See here the IDE’s the rust analyser supports.
Rust Rover–
IDE by Jet Brains – here. This is free for non-commercial users.
References-
https://doc.rust-lang.org/book/ch01-01-installation.html#installing-rustup-on-linux-or-macos
0 Comments
1 Pingback