Last Updated on April 14, 2023 by sandeeppote
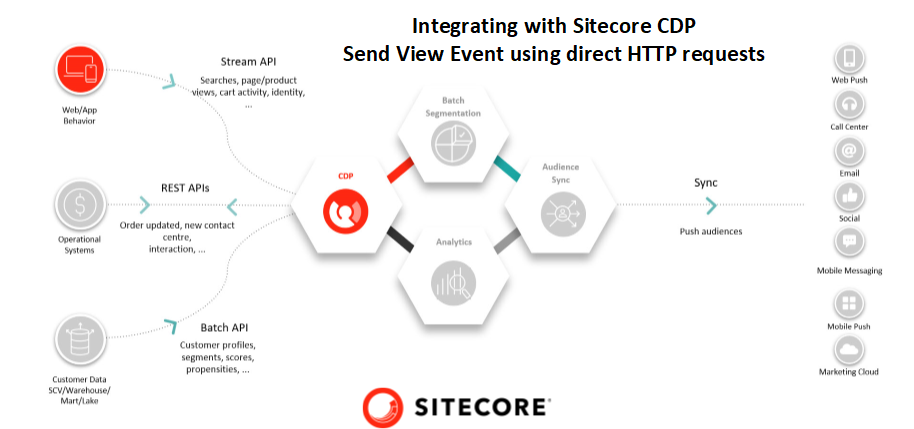
The VIEW event captures the guest’s action of viewing a page. To track guest behavior use VIEW event on all pages.
Sending a request through postman- use below URL-
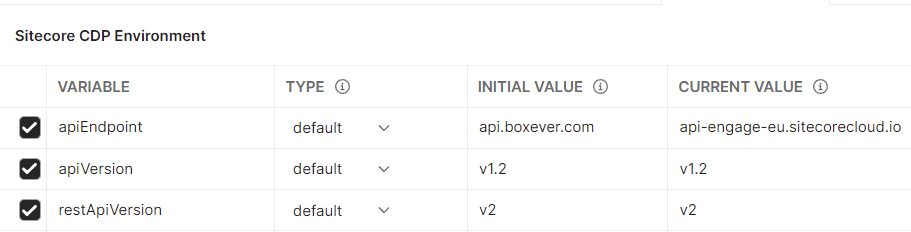
To know more what should be the API endpoint and api version see this blog
Generate browser id – see how to generate browserid using postman here
URL – contains event to create view event.
Message-
var browser_id = pm.environment.get("browserId")
var pointOfSale = pm.environment.get("PointOfSale")
var message = {
"channel": "WEB",
"type": "VIEW",
"language": "EN",
"currency": "GBP",
"page": "home page",
"pos": pointOfSale,
"browser_id": browser_id
}
postman.setEnvironmentVariable("message", JSON.stringify(message));
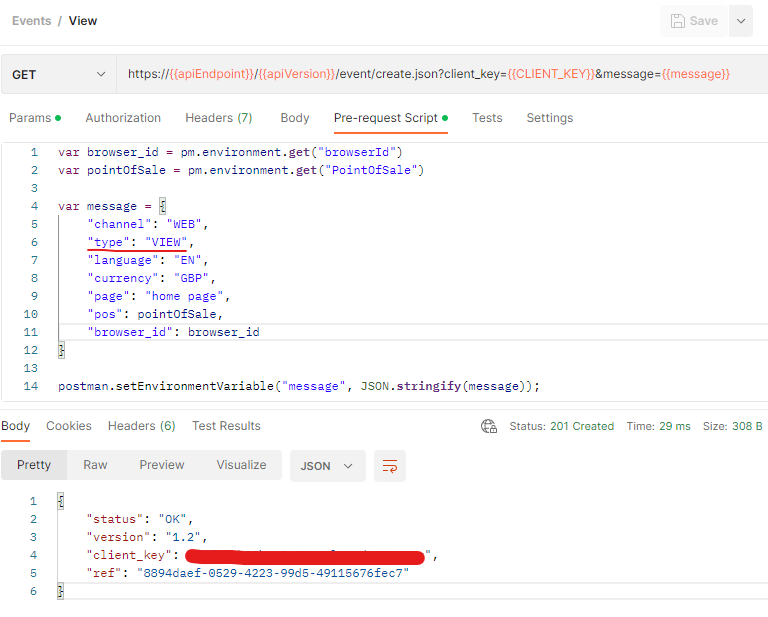
Check the view event in Sitecore CDP portal-
Search the Broswer Id in guests page-
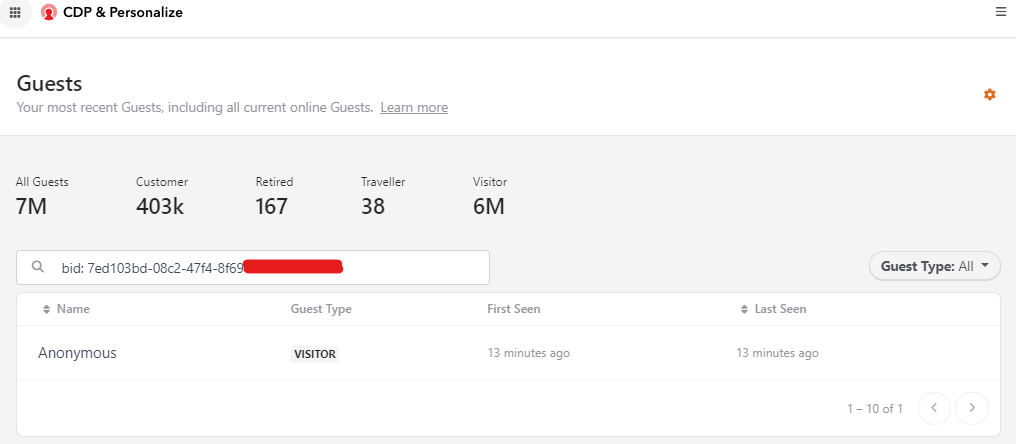
Select the Anonymous Visitor.
Select the Timeline tab
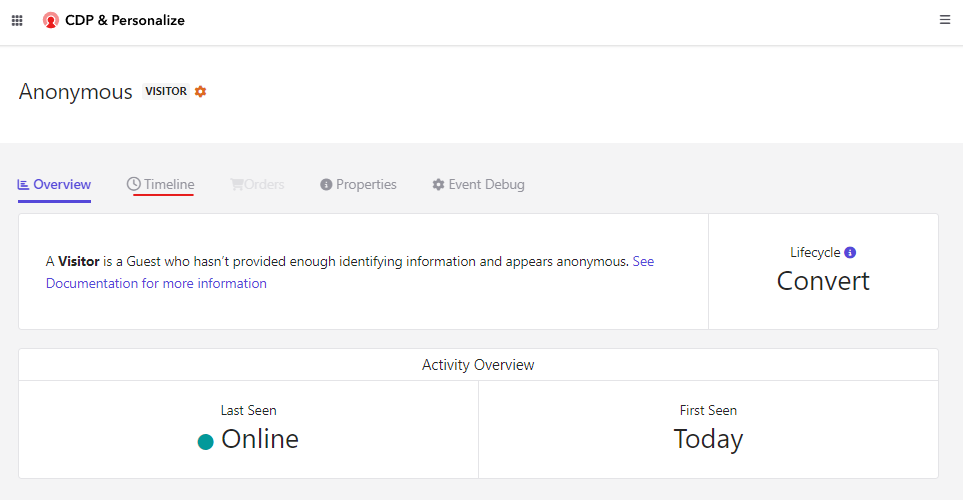
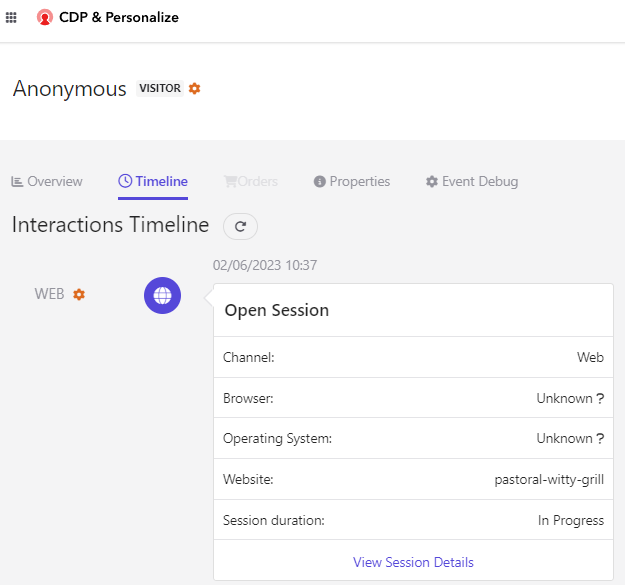
Select the orange color settings icon to check the view event details which is only displayed in debug mode-
See the detials the message that was sent as part of request-
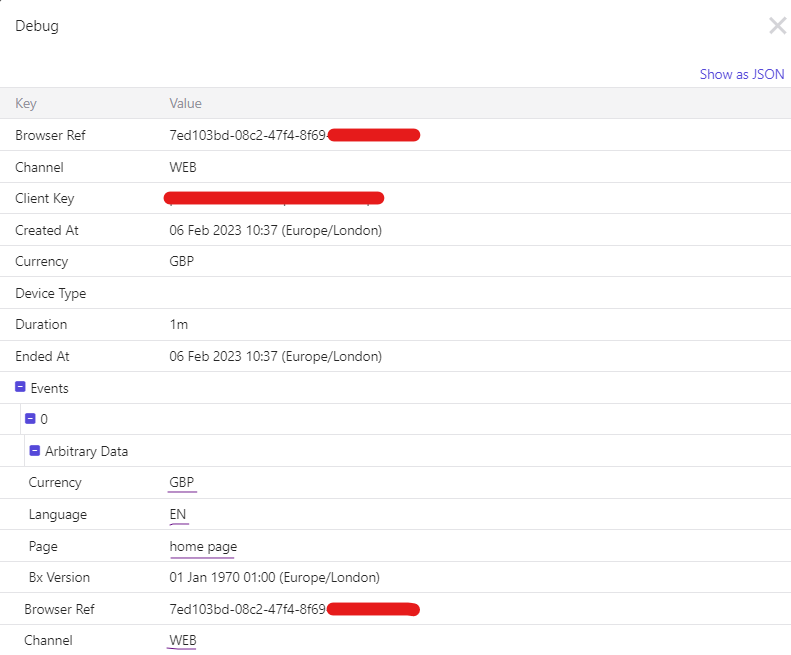
CURL command for same –
curl --location -g --request GET 'https://api-engage-eu.sitecorecloud.io/v1.2/event/create.json?client_key=<<enter client key>>&message={"channel":"WEB","type":"VIEW","language":"EN","currency":"GBP","page":"home page","pos":"pastoral-witty-grill","browser_id":"<<enter browser id>>"}'
C# code-
var client = new RestClient("https://api-engage-eu.sitecorecloud.io/v1.2/event/create.json?client_key=<<enter client key>>&message={\"channel\":\"WEB\",\"type\":\"VIEW\",\"language\":\"EN\",\"currency\":\"GBP\",\"page\":\"home page\",\"pos\":\"pastoral-witty-grill\",\"browser_id\":\"<<enter browser id>>\"}");
client.Timeout = -1;
var request = new RestRequest(Method.GET);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
Python- http client code snippet-
import http.client
conn = http.client.HTTPSConnection("api-engage-eu.sitecorecloud.io")
payload = ''
headers = {}
conn.request("GET", "/v1.2/event/create.json?client_key=<<enter client key>>&message=%7B%22channel%22:%22WEB%22,%22type%22:%22VIEW%22,%22language%22:%22EN%22,%22currency%22:%22GBP%22,%22page%22:%22home%20page%22,%22pos%22:%22pastoral-witty-grill%22,%22browser_id%22:%22<<enter browser id>>%22%7D", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Reference-