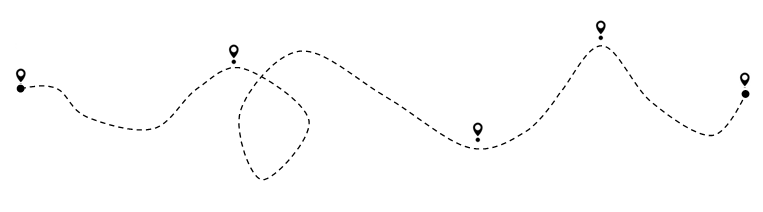
Routing constraints lets you restrict how the parameters in the route template are matched. It helps to filter out the input parameter and action method can accept.
For example if the URL Parameter is restricted to have int value, the route engine will match the controller action having integer value in the parameter or restrict.
How Route Constraints are applied-
- Using constraint parameter in the MapControllerRoute at the application startup where the endpoints are defined i.e. Inline Constraint
- Route attribute at the controller or action method
endpoints.MapControllerRoute(
name: "default",
pattern: "controller=Home}/{action=Index}/{id:int?}");
Route engine when matches the incoming url, it invokes the routing constraint to the the values in the url matches the pattern. In this case which is seperated by : in the above example restricts the value should be int or null.
Quick example on how the routing constraints are defined and used-
- Inline Constraint
Inline constraint are added after the URL parameter and sperated by : (colon) with the primitive type and also defines if the parameter can be nullable. Below code see – {id:int?}
app.UseEndpoints(
endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id:int?}");
}
);
The int constraints checks if the parameter value sent is integer and allows to execute the action method by passing the parameter value to the matching method.
The other way to define same if to pass the default values and constraint parameter in the MapControllerRoute
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: " {controller}/{action}/{id?}", new {Controller = "Home", action="Index"}, new {id = new IntRouteConstraint()});
}
);
- Constraints in route attribute
You can also define the constraints in route attribute as follows-
[Route("Home/Index/{id:int?}")]
public IActionResult Index(int? id)
{
return View();
}
Here are the list of constraints from the Microsoft doc site, try it yourself-
Constraint | Description | Example |
---|---|---|
alpha | Matches uppercase or lowercase Latin alphabet characters (a-z, A-Z) | {x:alpha} |
bool | Matches a Boolean value. | {x:bool} |
datetime | Matches a DateTime value. | {x:datetime} |
decimal | Matches a decimal value. | {x:decimal} |
double | Matches a 64-bit floating-point value. | {x:double} |
float | Matches a 32-bit floating-point value. | {x:float} |
guid | Matches a GUID value. | {x:guid} |
int | Matches a 32-bit integer value. | {x:int} |
length | Matches a string with the specified length or within a specified range of lengths. | {x:length(6)} {x:length(1,20)} |
long | Matches a 64-bit integer value. | {x:long} |
max | Matches an integer with a maximum value. | {x:max(10)} |
maxlength | Matches a string with a maximum length. | {x:maxlength(10)} |
min | Matches an integer with a minimum value. | {x:min(10)} |
minlength | Matches a string with a minimum length. | {x:minlength(10)} |
range | Matches an integer within a range of values. | {x:range(10,50)} |
regex | Matches a regular expression. | {x:regex(^\d{3}-\d{3}-\d{4}$)} |