Ownership in Rust where the variables are responsible for creating their own resources.
Every value is owned by one, and only one variable at a time
When the owning variable goes out of scope, the value is dropped
Memory Management in Rust and other languages
Memory Management | Feature | Advantage | Disadvantage |
Manual Allocation and Deallocation | Progammer responsible for memory mgmt C/C++ – malloc() and free() | Programmer has lots of control | Memory leaks |
Garbage Collection | Automatically cleans up memory Java, Python, C#, Ruby, Go | Its easy as programmer don’t have to worry about memory mgmt | Can lead to wasteful memory Can run at inconvenient times |
Ownership | Variables are responsible for freeing their own resources Rust | Safe, Effecient (when data will be dropped at compile time) | Requires understanding of ownership |
Example – Every value is owned by one, and only one variable at a time
{
1let inner_variable = String::from("Welcome");
println!("inner_valriable is {}", inner_variable);
}2
println!("inner_valriable is {}", inner_variable);3 // Error
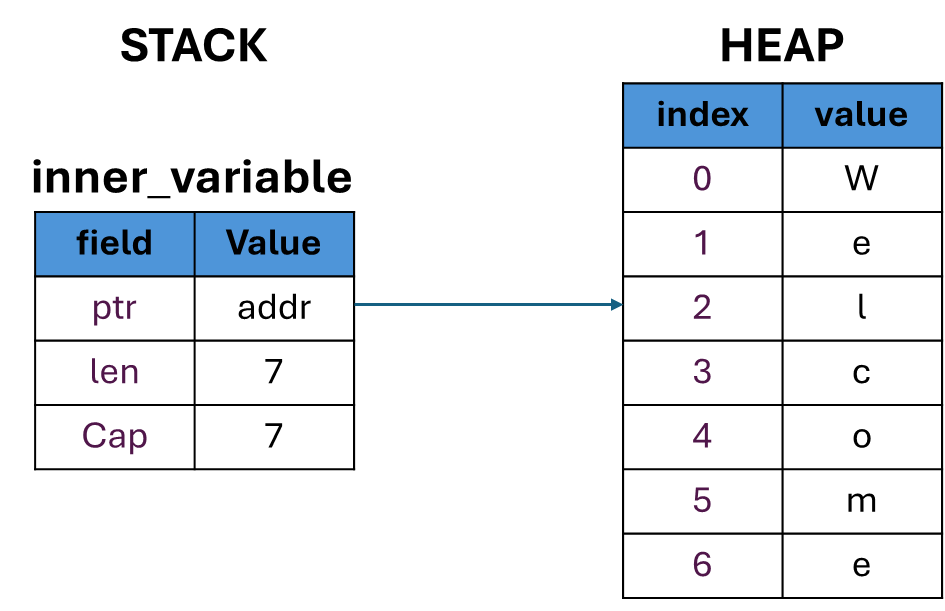
- The memory is allocated at this line of code in Stack and points to heap where the data is stored. ↩︎
- Deallocates/drops the data in heap and stack since the scope is complete ↩︎
- Error – Variable is nto available here since its deallocated in prevous line. ↩︎
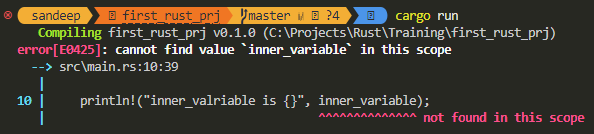
Example – When the owning variable goes out of scope, the value is dropped
Ownership is moved to another variable (outer_variable)
let outer_variable : String;
{
let inner_variable = String::from("welcome");
println!("inner_valriable is {}", inner_variable);
outer_variable = inner_variable;
}
println!("outer_variable is {}", outer_variable);
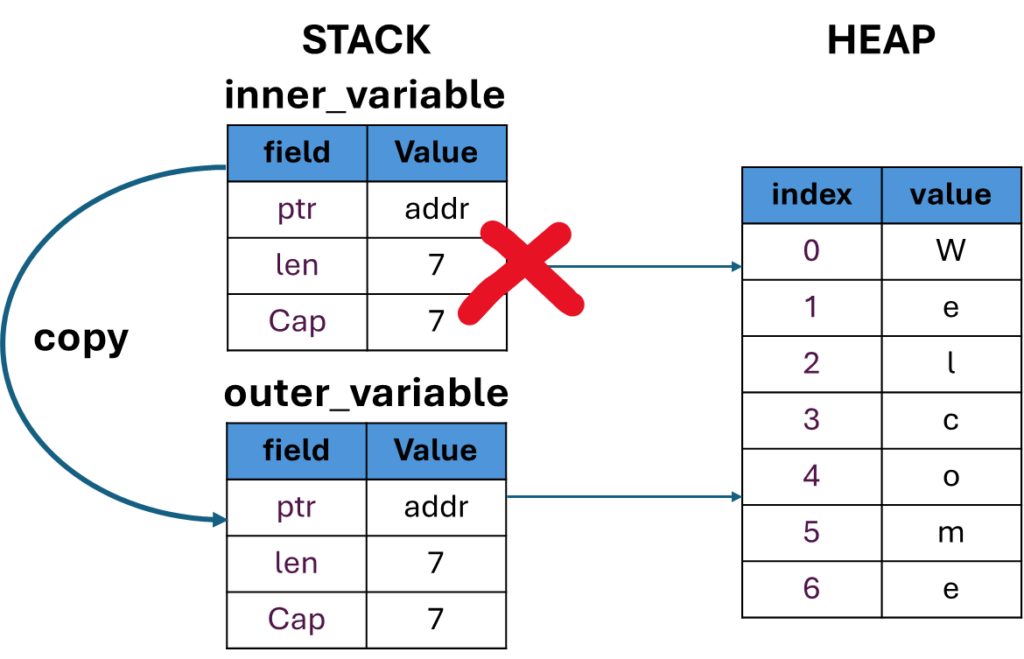
X The above example breaks the rule since 2 variables assigned to same address in heap. Hence this is called as move the inner_varaible is out of scope.
let outer_variable : String;
{
let inner_variable = String::from("welcome");
outer_variable = inner_variable;
println!("inner_valriable is {}", inner_variable); // Error
}
println!("outer_variable is {}", outer_variable);
Since the inner_variable scope is moved to outer_variable the memory allocation is dropped.
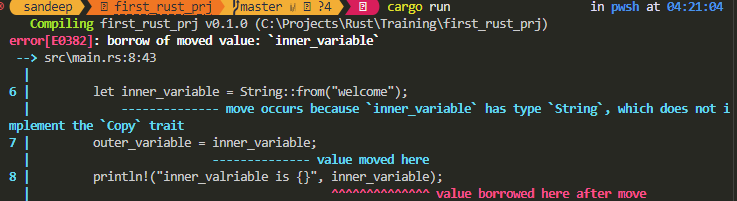
Copy works only for stack data types, such as integer and floating point
Cloning the data
The clone() method is used to create a deep copy of heap data.
String data is not implicitly copied and must be explicitly cloned using the clone() method.
let outer_variable : String;
{
let inner_variable = String::from("welcome");
outer_variable = inner_variable.clone();
println!("inner_valriable is {}", inner_variable);
}
println!("uter_valriable is {}", outer_variable);
Clone creates a new heap and copies the data and binds to the stack.
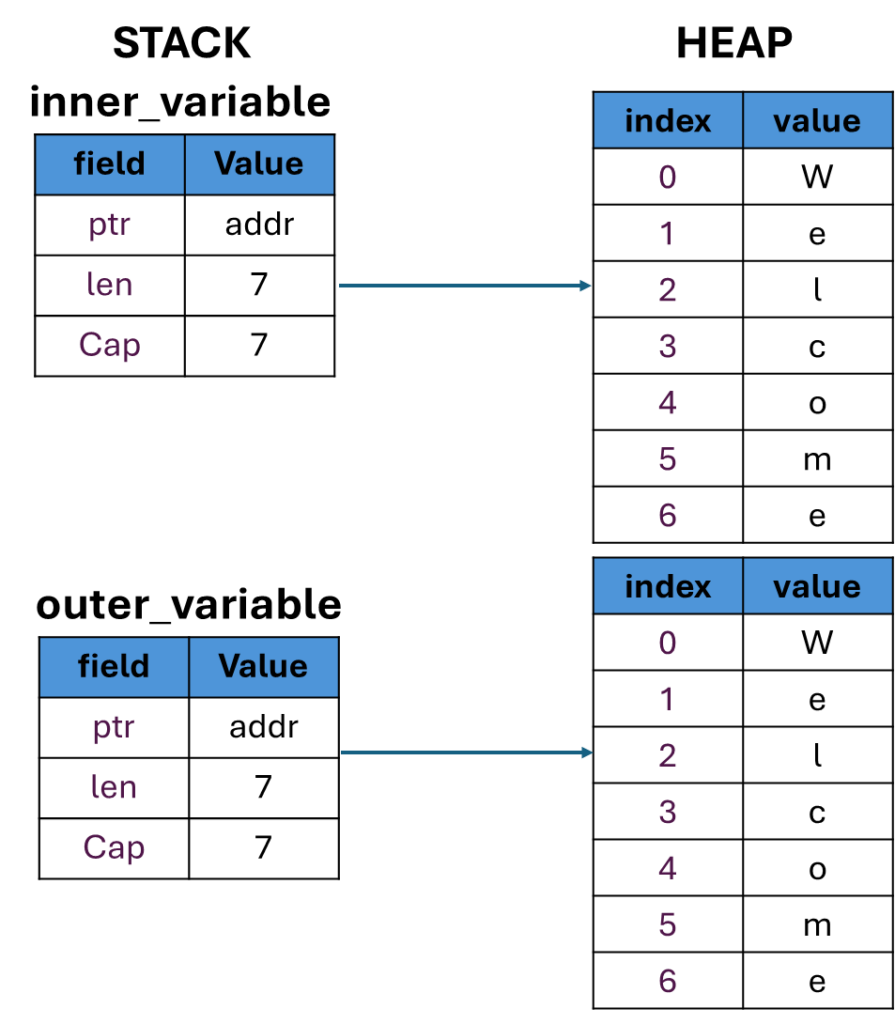
Output
inner_valriable is welcome
outer_valriable is welcome
Copy occurs implicitly; cloning must be done explicitly
Transfering Ownership
When a function takes ownership of a value, it becomes responsible for that value and its associated data. The function can then do whatever it wants with the data, including modify or delete it. If the function doesn’t need the data anymore, it will be dropped.
Hence Rust is Safe i.e. at compile time the program knows when the variables are accessible and efficient since it knows when to drop the data from memory.
Summary-
Shadowing allows you to declare a new variable with the same name as an existing variable.
Copying allows you to duplicate a value that is stored on the stack.
The heap can dynamically add and remove data.
The stack stores values in sequential order. The stack adds and removed data as LIFO.
Copying data on the stack occurs implicitly, whereas cloning data on the heap must be done explicitly using the clone method.
The String data type stores the sequence of characters that make up the String on the heap and it stores a structure containing a pointer to the heap data, the String’s length, and its capacity on the stack.
Rust uses an ownership model to manage memory.