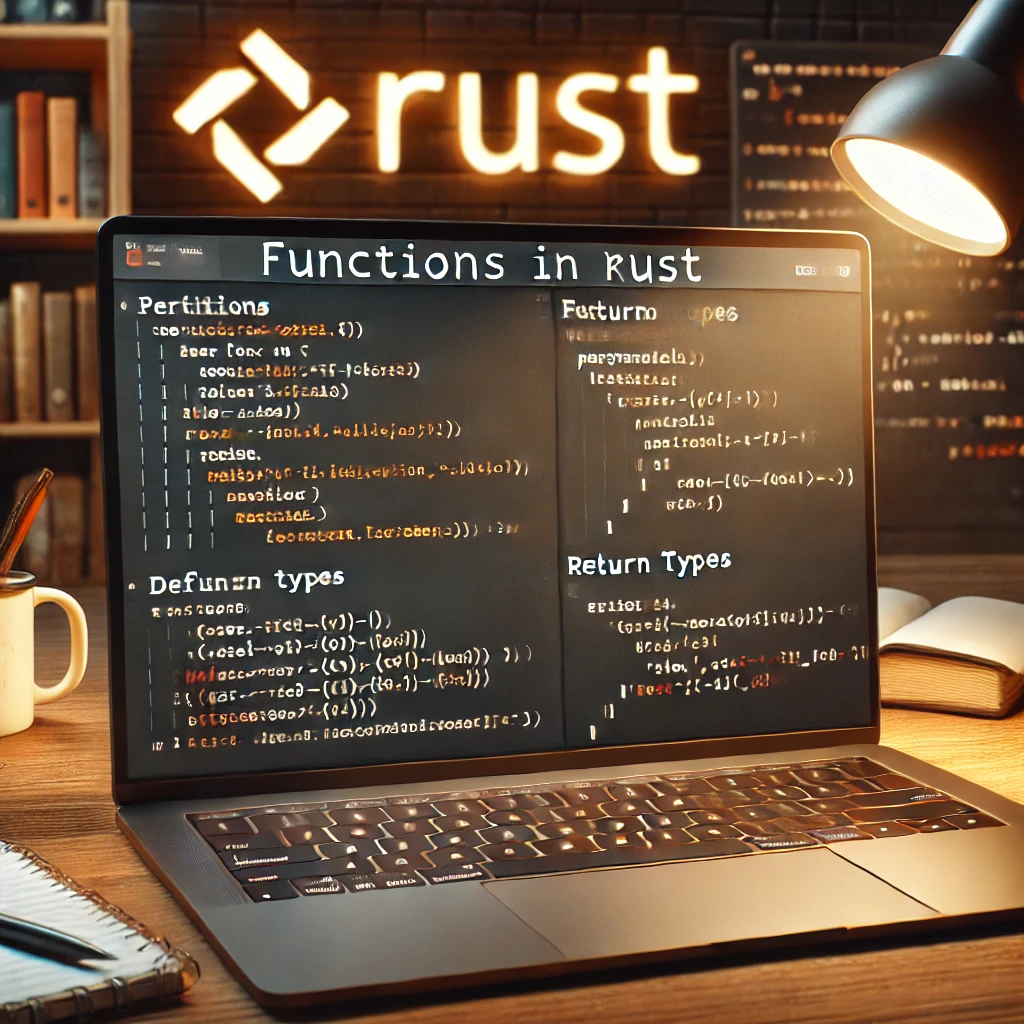
Functions are use for efficient programming and eliminates the need to constantly rewrite the code.
Rust is a strictly typed language and therefore needs to know the data type for all variables at compilation.
When there is not a specified return value, the function will return the unit data type which is represented as () and indicates there is no other meaningful values that could be returned. A statement does not return a value.
In the below example we can see how a function can be defined and called from the main.
Function without and with parameters. Define the parmeter data type and return data type.
fn main(){
display_message();
display_message_with_parameters(10);
let addition = add_numbers(10, 20);
println!("Addition of 2 number is {}", addition);
}
fn display_message(){
println!("Display message....");
}
fn display_message_with_parameters(value : i8){
println!("Function paramter value is {}", value);
}
fn add_numbers(param1: i8, param2: i8) -> i8{
param1 + param2
}
Output-
Display message....
Function paramter value is 10
Addition of 2 number is 30
Diverging Functions
Diverging functions never return. They are marked using !
, which is an empty type.
fn foo() -> ! {
panic!("This call never returns.");
}
Empty return functions
Function return empty (). It doesn’t return anything but returns back to caller
fn some_fn() {
()
}
fn main() {
let _a: () = some_fn();
println!("This function returns and you can see this line.");
}