Last Updated on May 17, 2025 by sandeeppote
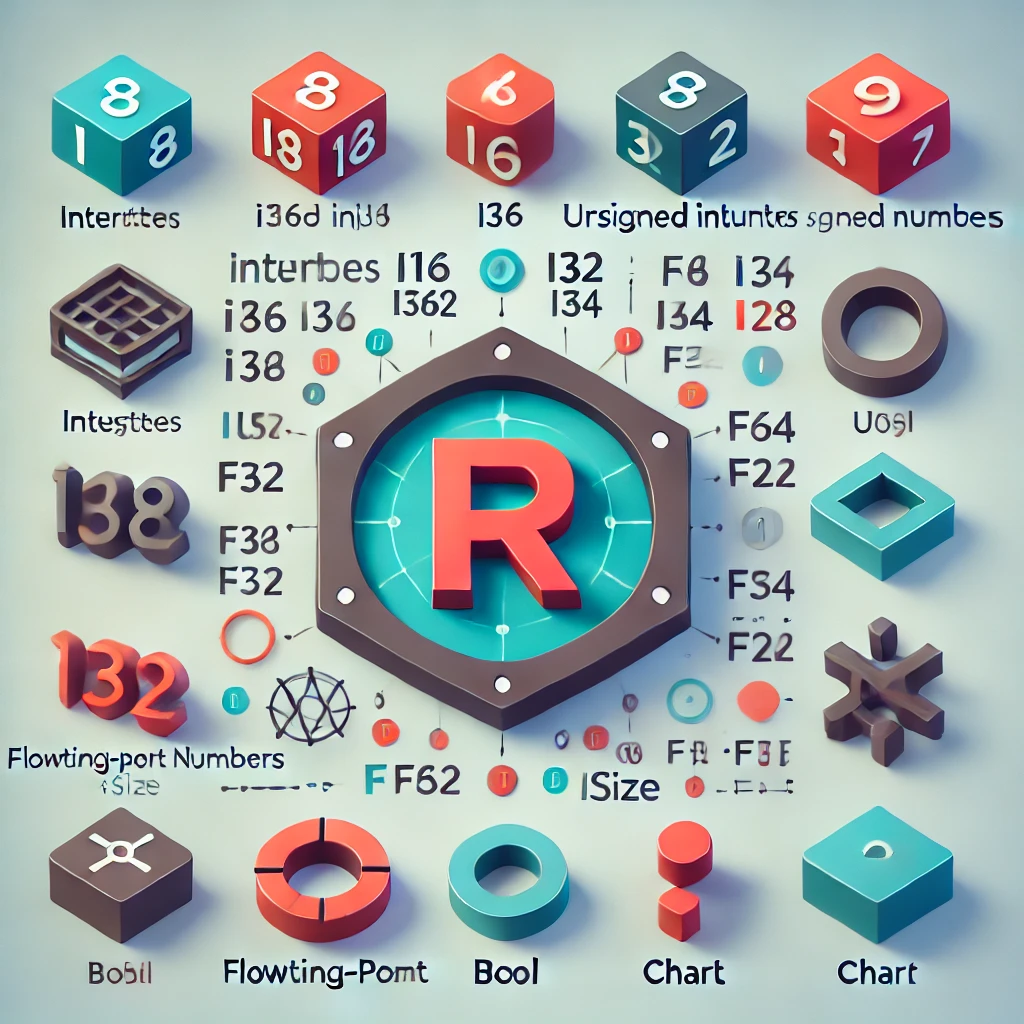
Rust variables are immutable by default.
Variables must be explicitly declared as mutable using mut keyword
Rust is a statically typed language – all variable data types must be know at compile time.
Example-
//default immutable. value cannot be changed after declaration
let x = 10;
// marked as mutable. i.e value can be changed after declaration
let mut y = 20;
Compiler Errors-
let x = 10;
println!("x is {}", x);
x=20; // Error. Cannot be assigned twice. consider making this muttable
println!("x is {}", x);
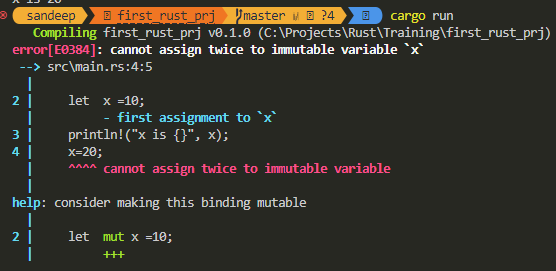
Solution-
let mut x = 10; // make this mutable
println!("x is {}", x);
x=20; // compiles successfully
println!("x is {}", x);
Reference –
https://doc.rust-lang.org/1.0.0/style/style/naming/README.html
https://rust-lang.github.io/api-guidelines/naming.html
Integer Data Types–
Reference – https://doc.rust-lang.org/reference/types/numeric.html
- Unsigned – only represent positive values
- Signed – represent positive and negative values
- Characterized by number of bits
Length | Signed | Unsigned |
---|---|---|
8-bit | i8 ( -(27) to 27-1) | u8 ( 0 to 28-1) |
16-bit | i16 ( -(215) to 215-1) | u16 ( 0 to 216-1) |
32-bit | i32 ( -(231) to 231-1) default | u32 ( 0 to 232-1) |
64-bit | i64 ( -(263) to 263-1) | u64 ( 0 to 264-1) |
128-bit | i128 ( -(2127) to 2127-1) | u128 ( 0 to 2128-1) |
Type of the varaible is declared after the : (colon)
Example:-
// signed 8-bit integer. max value can hold is 255
let x: i8 = 255;
let y: i8 = -10;
// unsigned 8-bit integer.
let z: u8 = 255;
Compiler Errors-
// unsigned 8-bit integer. max value 255
let z: u8 = 256;
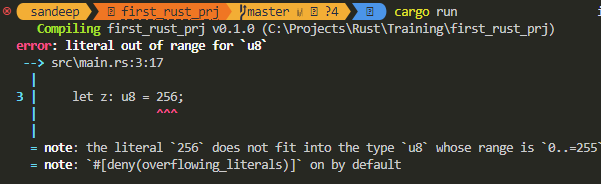
Solution-
// unsigned 8-bit integer. max value 255
let z: u8 = 255; // compiles successfully
Runtime Errors-
// unsigned 8-bit integer. max value 255
let mut z: u8 = 255;
z = z + 1; // Error: panicked - attempt to add with overflow
println!("z is {}", z);
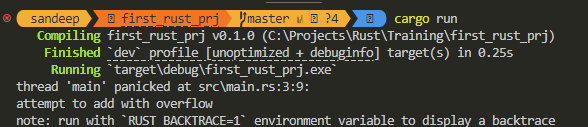
Solution-
// unsigned 8-bit integer. max value 255
let mut z: u8 = 254;
z = z + 1; // compiles and runs successfully
println!("z is {}", z);
Output- z is 255
Floating-Point Data Types
- Represent numbers with decimal points
- f32 and f64 are two floating-point types
- Value stored as fractional and exponential components
f32- represents value from 6 to 9 decimal digits of precision.
f64- represents value from 15 to 17 decimal digits of precision.
Example-
let x= 10.00; // default is f64
println!("x is {} deafult type is f64", x);
let y: f32= 10.1234567890132456798; // default is f64
println!("y is {} with f32 floating-point type", y);
let z: f64= 10.1234567890132456798; // default is f64
println!("z is {} with f64 floating-point type", z);
Output-
x is 10 deafult type is f64
y is 10.123457 with f32 floating-point type
z is 10.123456789013245 with f64 floating-point type
Guidelines for Numeric data types–
Values with fractional component or decimal places use floating-point data type.
Use f64 or been default gives most precision range. With modern computers it is as good as using f32. So perrfomance should not be an issue.
Use f32 with embedded systems where memory is a limitation.
Default 32 signed integer (i32) provides a generous range – between and around plus or minus 2 billion
For memory concerns use smaller size integers to conserve and use less memory
Arithmetic Operations
Rust uses arithmetic operations with the operators +, -, *, / and %
Artihmentic operation results depend on the type of variables. example, if both operators are integer then will get result in integer
let x= 10; // default is i32
let y=3; // default is i32
let z= x / y;
println!("z is {}", z) // so z will be i32
Output- Here the value of z is 3 since both the variables are defaulted to signed integer i.e. i32
z is 3
Now if we add decimals to x and y the output is floating-point-
let x= 10.00; // default is f64
let y=3.00;
let z= x / y;
println!("z is {}", z)
Output- Here the default is f64.
z is 3.3333333333333335
Compiler Errors-
If one of the variables data type is different from other, then will receive error-
let x= 10; // default is i32
let y=3.00; // default is f64s
let z= x / y;
println!("z is {}", z)
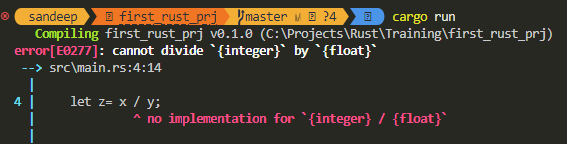
Here the default integer is i32 and floating-point is f64. Dividing different data types will result in error. This is applicable for all artihmetic operations.
Solution-
For the above error cast the variable.
let x= 10; // default is i32
let y=3.00; // default is f64s
let z= x as f64 + y;
println!("z is {}", z)
Output-
z is 3.3333333333333335
Formatting print statements
Reference- https://doc.rust-lang.org/std/fmt/
To format the data use Module fmt. This uses the formatting extensions.
Use {} as argument to print the values
Examples-
println!("Hello {}!", "world"); // prints- Hello world!
//named parameters
println!("{value}", value=10); // prints- 10
// named paarameters using variable
let name = "Sandeep Pote";
println!("Name: {name}");
// Decimal point upto 3
let z= 3.33333335;
println!("z is {:.3}", z); // prints - 3.333
// Upto 3 decimal places with lenth of 8 and preceding 0's
println!("z is {:08.3}", z); // prints- 0003.333
Output-
Hello world!
10
Name: Sandeep Pote
z is 3.333
z is 0003.333
Positional Parameters
Here the first_name and last_name are named parameters, but the position of printing the parameters are set in different order
println!("{last_name} {first_name}", first_name="Sandeep", last_name="Pote"); //Prints- Pote Sandeep
Binary
Binary trait formats the output as a number in binary
Example-
let value= 0b11110101u8;
println!("value is {}", value)// Prints the number
println!("value is {:b}", value) // prints the binary
println!("value is {:010b}", value) // prints the binary upto 10 digits and preceding 0.
Output-
value is 245
value is 11110101
value is 0011110101
Here the binary is converted to number. The prefix 0b mentions the value is binary and suffix u8 converts the binary to unsigned integer.
Bitwise operations-
Logical operations on patterns using NOT, AND, OR, XOR and SHIFT.
Taking the above example, Bitwise NOT-
let mut value= 0b11110101u8;
value=!value;
println!("value is {:08b}", value); // prints the negated binary
Output-
value is 00001010
Bitwise AND- change the value of a specific Bit-
Use the & with the anotehr binary to change the value.
Example- to change the value of the highlighted 11110111 to 0, & this with 11110011. Ouput will be 11110011
Example-
let mut value= 0b11110111u8;
value=value & 0b11110011;
println!("value is {:08b}", value);
Output-
value is 11110011
Likewise use | (pipe) for OR, ^ for XOR, << Left shift and >> Right Shift
Boolean data types and operations
Use the above operatos used in bitwise for boolean operations.
Example-
let a = true;
let b= false;
println!("a is {} and b is {}", a, b);
println!("NOT a is {} ", !a);
println!("a AND b is {} ", a & b);
println!("a OR b is {} ", a | b);
println!("a XOR b is {} ", a ^ b);
Output-
a is true and b is false
NOT a is false
a AND b is false
a OR b is true
a XOR b is true
Short-Circuiting Logical Operations
With this in OR operation if the left side is True then it won’t check the right side since the result for OR will be always true.
Similarly, for AND operation if the left side is False, it won’t check the value at the right side, since the result will be always False.
This can be done using && or ||. This improves the code effeciency.
Example-
let a = true;
let b= false;
let c= (a ^ b) || (a & b);
println!("c is {}", c);
Output-
c is true // (a ^ b) is true || (a & b) is false
Comparision Operations
Reference – https://doc.rust-lang.org/book/appendix-02-operators.html
Use compareision operators to compare values. Some of these are-
- Equal ==
- Not Equal !=
- Greater Than >
- Less Than <
- Greater Than Equal >=
- Less Than Equal <=
Example-
let a = 1;
let b= 2;
println!("a is {} and b is {}", a, b);
println!("a EQUAL TO b is {} ", a == b);
println!("a NOT EQUAL TO b is {} ", a != b);
println!("a GREATER THAN TO b is {} ", a > b);
println!("a LESS THAN TO b is {} ", a < b);
println!("a GREATER THAN EQUAL TO b is {} ", a >= b);
println!("a LESS THAN EQUAL TO b is {} ", a <= b);
Output-
a is 1 and b is 2
a EQUAL TO b is false
a NOT EQUAL TO b is true
a GREATER THAN TO b is false
a LESS THAN TO b is true
a GREATER THAN EQUAL TO b is false
a LESS THAN EQUAL TO b is true
The comparision can be used with the similar data types.
Char Data Type
- Represents a single character
- Stored using 4 bytes
- Unicode scalar value
Char can be represented within a single quote e.g.- ‘a’
Unicode characters can be represented using “\u{hexa decimal value}”
Reference- https://home.unicode.org/
Example-
let letter = 'a';
let number = '1';
let unicode= '\u{0024}';
println!("Letter is {}", letter);
println!("Number is {}", number);
println!("Unicode is {}", unicode);
Output-
Letter is a
Number is 1
Unicode is $
0 Comments
1 Pingback